custom dialog๋ ์๋๋ก์ด๋๋ฅผ ๊ฐ๋ฐํ๋ฉด์ ์์ฃผ ์ฐ์ด๋ ํ์ ์ฐฝ์ ์์ฑํด์ฃผ๋ ๊ธฐ๋ฅ์ด๋ค.
ํ์๋ ๋ง์ด ์์ฃผ ์ฐ์ง๋ง, ๋ง์ด ๊น๋จน๊ธฐ(?) ๋๋ฌธ์ ์ด๋ฒ์ ๊ธฐ๋ก์ ๋จ๊ฒจ๋ณด๋ ค ํ๋ค.
custom dialog ๋ง๋๋ ๋ฐฉ๋ฒ
1. custom dialog๋ก ์ฌ์ฉํ xml ํ์ผ ๋ง๋ค๊ธฐ
๋จผ์ dialog์ xml๋ก ๋ ์ด์์์ ๋ง๋ค์ด ์ค๋ค.
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:orientation="vertical">
<ImageView
android:id="@+id/title_image"
android:layout_width="150dp"
android:layout_height="150dp"
android:layout_marginTop="20dp"
android:src="@drawable/addimage"
android:layout_gravity="center"/>
<LinearLayout
android:id="@+id/chat_info_layout"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:layout_marginTop="20dp"
android:layout_marginStart="30dp"
android:layout_marginEnd="30dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/title_image">
<TextView
android:id="@+id/name"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:fontFamily="@font/dalseohealingbold"
android:text="์ด๋ฆ: "
android:textSize="25sp"
android:layout_marginEnd="10dp"/>
<EditText
android:id="@+id/chatting_room_edit_text"
android:layout_width="230dp"
android:layout_height="60dp"
android:background="@color/white"
android:fontFamily="@font/dalseohealingbold"
android:hint="์ฑํ
๋ฐฉ ์ด๋ฆ"
android:maxLines="1"
android:textSize="22sp" />
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginStart="50dp"
android:layout_marginTop="20dp"
android:layout_marginEnd="50dp"
android:layout_marginBottom="20dp"
android:orientation="horizontal">
<androidx.appcompat.widget.AppCompatButton
android:id="@+id/create_chatting_room"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="@color/greenyellow"
android:fontFamily="@font/dalseohealingbold"
android:text="์์ฑ" />
<View
android:layout_width="0dp"
android:layout_height="0dp"
android:layout_weight="1" />
<androidx.appcompat.widget.AppCompatButton
android:id="@+id/cancel_create"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="@color/greenyellow"
android:fontFamily="@font/dalseohealingbold"
android:text="์ทจ์" />
</LinearLayout>
</LinearLayout>
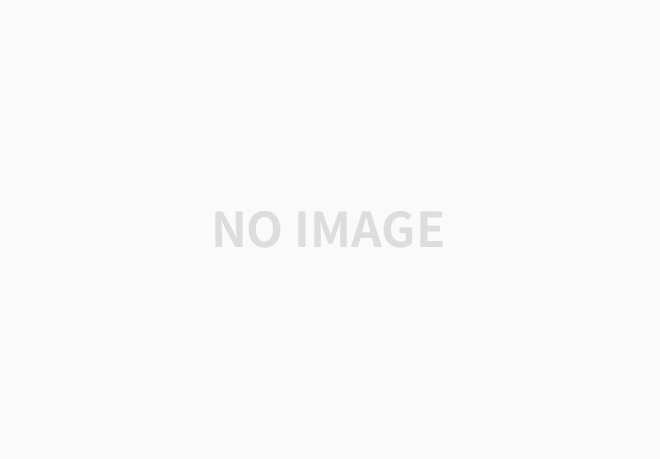
2. ๋ค์ด์ผ๋ก๊ทธ ์์ฑํ๊ธฐ
์ผ๋จ ํ์๋ ๋ค์ด์ผ๋ก๊ทธ๋ฅผ ๋ทฐ ๋ฐ์ธ๋ฉ์ผ๋ก ๊ตฌํ ํ ๊ฒ์ด๊ธฐ ๋๋ฌธ์ ๊ทธ๋๋ค์ ์ถ๊ฐํด์ฃผ์๋ค.
viewBinding {
enabled = true
}
๊ทธ ๋ค์์ ๋ค์ด์ผ๋ก๊ทธ๋ฅผ ๊ตฌํํ๋ค.(ํ์๋ fragment์ ๋ค์ด์ผ๋ก๊ทธ๋ฅผ ๊ตฌํํ๋ค.)
activity?.window?.setBackgroundDrawable(ColorDrawable(Color.TRANSPARENT))
val dialogBinding = DialogCreateRandomChattingBinding.inflate(layoutInflater)
val dialogBuilder = AlertDialog.Builder(context)
.setView(dialogBinding.root)
val dialog = dialogBuilder.show()
dialogLogic(dialogBinding, dialog) // ๋ค์ด์ผ๋ก๊ทธ์ ๊ธฐ๋ฅ์ ๋ด์ ํจ์
์คํ ๊ฒฐ๊ณผ
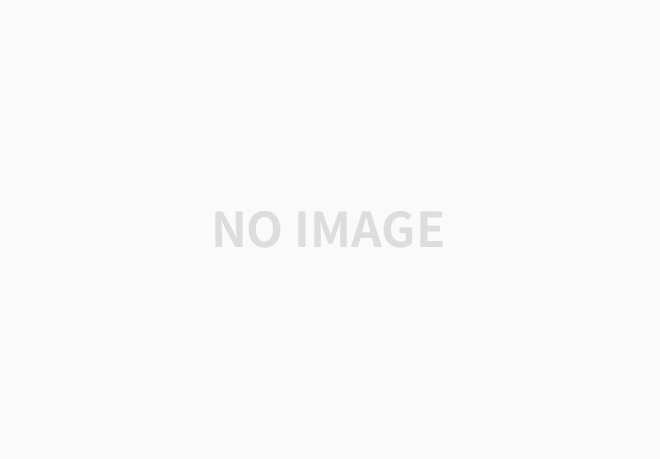
๋ค์ด์ผ๋ก๊ทธ๊ฐ ์ ์์ฑ์ด ๋์๋ค.
์ฝ๊ฐ์ tmi์ ๋จ๊ธฐ๋ฉด
custom dialog์ ๊ธฐ๋ณธ width, height๋ wrap_content ์ด๊ธฐ ๋๋ฌธ์ xml๋ก ์ง ๋ชจ์ต๊ณผ ๋ค๋ฅด๊ฒ ๋์ฌ ์ ์๋ค.๊ทธ๋์ ํ์๋ ๊ธฐ๋ณธ width, height๋ฅผ ๋ฐ๊ฟ ๋ฐฉ๋ฒ์ ๊ฒ์ํด ๋ณด์์ง๋ง ์ ๋์ค์ง ์์๋ค. ๊ทธ๋์ ๊ทธ๋ฅ xml์ wrap_content๋ฅผ ๊ธฐ์ค์ผ๋ก ํด์ ์ฝ๋๋ฅผ ์งฐ๋ค.
'๐ฑ| Android > ๐ | ๊ธฐ๋ก' ์นดํ ๊ณ ๋ฆฌ์ ๋ค๋ฅธ ๊ธ
[Android, Kotlin] Android MVVM ๋ค๋ค๋ณด๊ธฐ (1) | 2023.03.18 |
---|---|
[Android, Kotlin] Zxing ๋ผ์ด๋ธ๋ฌ๋ฆฌ๋ก QR์ฝ๋ ์ค์บํ๊ธฐ (5) | 2023.03.11 |
[Android, Kotlin] Android์์ Apollo๋ฅผ ์ด์ฉํ GraphQL์ฌ์ฉํ๊ธฐ (0) | 2022.10.23 |
[Android, Kotlin] editText์ toggle icon์ด ๋ฐ๋๋ก ๋์์ ๋ ํด๊ฒฐ ๋ฐฉ๋ฒ (2) | 2022.08.31 |
[Android/Kotlin] retrofit2 ์ ๋ฆฌ (0) | 2022.05.13 |